Python is a versatile programming language that you can use on the backend, front end, or full web application stack.
In this article, I will give you an overview of the way of removing Leading Zeros from Python String.
How to Remove Leading Zeros in Python
Leading zeros in Python can be removed using the int() method type casting as well as by using the lstrip() method and the startswith() method and reassigning.
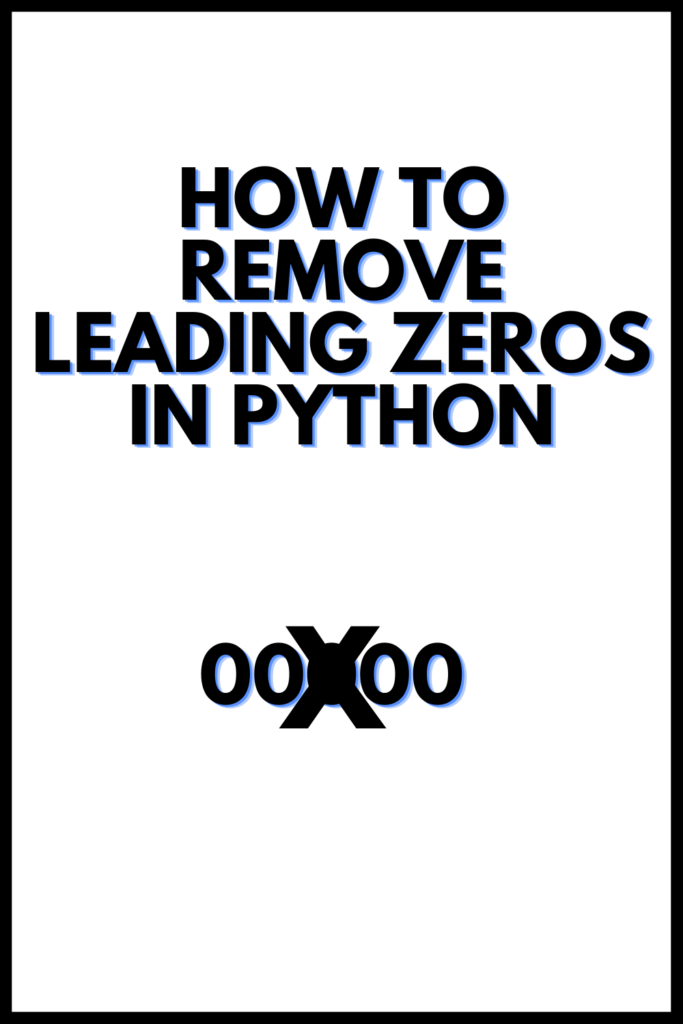
Leading Zeros in Python
Leading zeros are often used for padding purposes, such as in phone numbers or zip codes.
However, they can also lead to confusion and errors in your code if you’re not careful.
For example, the string “0001” could be interpreted as an integer value of 1,000, while the string “00001” would be interpreted as an integer value of 5.
In both cases, the leading zeros are unnecessary and can safely be removed without affecting the meaning of the data.
Therefore it is important to know the possible ways to remove the leading zeros in Python.
The below part of this article will talk about the methods of removing leading zeros in Python in more depth.
Removing Leading Zeros from a Python String
Using the int() Method/Type Casting in Python to Remove Leading Zeros
The first and naive method is the typecasting method using the int() function. The int() function comes with Python’s built-in library. It converts a string into an integer explicitly. The int() function is pretty easy to handle. You will simply pass your string through the function, and it will return a typecasted integer value. Please remember that your string cannot hold letters, alphabets, or other symbols while using the function. Here is an example for you.
my_customString = "00001357" my_customString = int(my_customString) print(my_customString)
Output: 1357
Using the lstrip() method to Remove Leading Zeros in Python
The lstrip() is Python’s most used function for removing leading zeros. This function also comes with Python’s in-built library. It eliminates the string given as the argument from the string in operation. The good thing about this function is it only removes the given string from the leading. Therefore, you can use it to remove the leading zeros from your python string.
Note: there is another function strip() that removes string from both leading and ending. So, use the functions as per your demand.
my_customString = "00001357" my_customString = my_customString.lstrip("0") print(my_customString)
Output: 1357
Using the startswith() Method and Reassigning to Remove Leading Zeros in Python
The startswith() function follows a check and proceeds formula. You can apply this function to your string through a loop. The startswith() function returns a boolean type.
Once you get your boolean result, you are up to running an iteration through the whole string to remove the leading zeros from your given string. Let me give you an example.
my_customString = "00001357" x = my_customString.startswith("0") print(x)
Output: True
Now you can impose an iteration this way.
my_customString = "00001357" for i in my_customString: if i == "0": my_customString = my_customString[1:] print(my_customString)
Output: 1357
Removing Leading Zeros from Python Integer
The above methods talk about removing leading zeros from Python strings. Now I’ll show you how to remove leading zeros from an integer.
For instance, let’s assume you have an ip address and want to vacate the zeros from the ip address. Generally, an ip address contains a couple of zeros in it. Your goal is to remove leading zeros from the ip address.
The approach is splitting the given ip address, converting it to an integer, and then rejoining it.
# Create a function to remove leading zeros def removeZerosFromIp(ip): ipWithoutZeros = ".".join([str(int(i)) for i in ip.split(".")]) return ipWithoutZeros; #Driver Code ip ="013.019.213.166" print(removeZerosFromIp(ip))
Output: 13.19.213.166
Removing Leading Zeros from a Python File Name
You might have files in your machine that contain leading zeros. For instance, 01.jpg, 03.txt, 09.docx, and you want to remove the leading zeros from your Python file name.
Yes, it is possible, and you can do it conveniently.
To strip the leading zeros from your file name, you have to import the os module in your Python script. Here is an example for better understanding.
import os, sys for filename in os.listdir(file folder): os.rename(filename, filename[1:])
In this article, I shed light on removing leading zeros in Python for different circumstances. I hope you’ll find it helpful.
Conclusion on How to Remove Leading Zeros in Python
To remove leading zeros in Python you can use:
- int() method type casting
- lstrip() method
- startswith() method reassigning